Mastering JavaScript URI Parsing for Web Design and Software Development
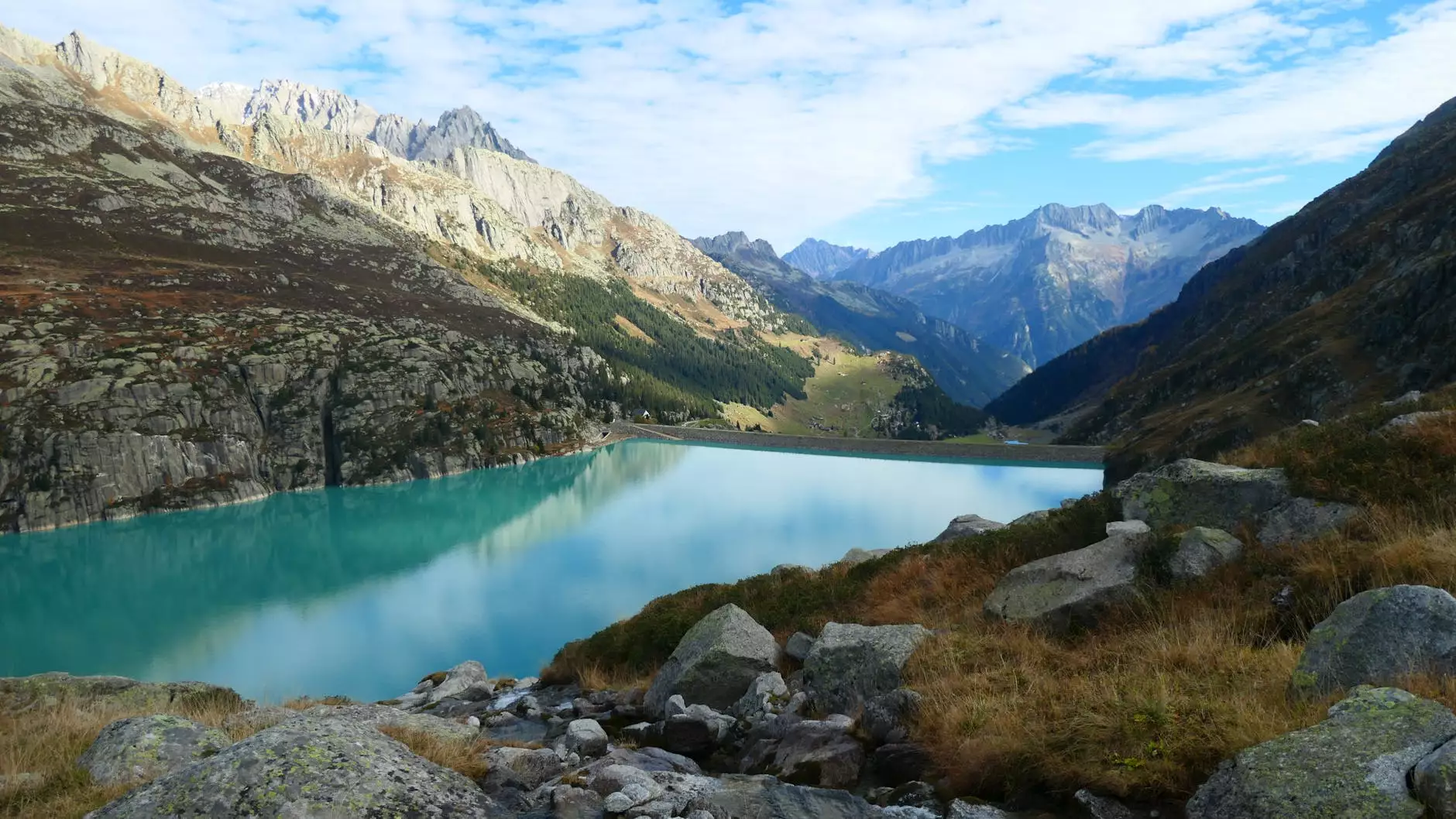
In the realm of web design and software development, parsing Uniform Resource Identifiers (URIs) is a fundamental skill that every developer should master. A thorough understanding of how to javascript parse uri can lead to better application performance, improved user experiences, and more efficient data handling. In this article, we will dissect the concept of URI parsing in JavaScript, delve into its significance, and provide practical examples that demonstrate its utility in real-world applications.
What is a URI?
Before we get into the intricacies of JavaScript URI parsing, it's essential to clarify what a URI is. A URI, or Uniform Resource Identifier, is a string of characters that uniquely identifies a particular resource on the internet. URIs come in two primary forms:
- URL (Uniform Resource Locator): Represents the location of a resource on the web.
- URN (Uniform Resource Name): Still identifies a resource but doesn't specify its location.
Importance of Parsing URIs
Parsing URIs is crucial in web and software applications for several reasons:
- Data Extraction: Developers can retrieve specific data from URIs to process web requests effectively.
- Routing: Many frameworks use URI segments to determine how to route requests to different application handlers.
- Security: By validating and sanitizing URL parameters, developers can protect their applications from common vulnerabilities.
How to JavaScript Parse URI
Now that we understand the fundamentals of URIs and their importance, let's dive into how we can javascript parse uri. JavaScript provides several ways to parse URIs effectively, primarily through the URL interface and regular expressions.
Using the URL Interface
The URL interface (available in modern browsers) makes parsing very straightforward. Here’s how you can use it:
const url = new URL('https://example.com:8080/path/to/resource?query=example#fragment'); console.log(url.protocol); // 'https:' console.log(url.hostname); // 'example.com' console.log(url.port); // '8080' console.log(url.pathname); // '/path/to/resource' console.log(url.search); // '?query=example' console.log(url.hash); // '#fragment'In this example, we create a new URL object, which automatically parses the provided URI. You can easily access different components of the URI, which simplifies various operations, such as redirection and fetch requests.
Regular Expressions for URI Parsing
If you're working in an environment where the URL interface isn’t available, or for more customized parsing requirements, regular expressions can be a valuable tool. Below is an example of how you can use regex to extract pieces of a URI:
const uri = 'https://example.com:8080/path/to/resource?query=example#fragment'; const regex = /^(https?:\/\/)?([^\/:]+)(:\d+)?(\/[^?#]*)?(\?[^#]*)?(#.*)?$/; const matches = uri.match(regex); const protocol = matches[1] || 'http'; const hostname = matches[2]; const port = matches[3] ? matches[3].substring(1) : '80'; const pathname = matches[4] || '/'; const search = matches[5] || ''; const hash = matches[6] || ''; console.log('Protocol:', protocol); console.log('Hostname:', hostname); console.log('Port:', port); console.log('Pathname:', pathname); console.log('Search:', search); console.log('Hash:', hash);Regular expressions allow for greater customization but can be complex to maintain. Ensure you have a solid grasp of regex if you choose this method.
Common Use Cases for URI Parsing
URI parsing is not just a theoretical exercise; it has numerous practical applications in web design and software development:
1. Dynamic URL Management
Many modern web applications require dynamic URL management. For instance, a blog application might need to generate URLs based on the article's title. By parsing and manipulating URIs, developers can create user-friendly URLs that enhance SEO and usability.
2. API Interaction
When developing applications that consume APIs, understanding how to javascript parse uri is critical. Parameters in a query string may affect API requests, and parsing them correctly ensures accurate data retrieval.
3. Analytics and Tracking
Effective tracking of user behavior often relies on URIs. By extracting and analyzing query parameters, businesses can tailor their marketing strategies and improve customer engagement.
4. Form Handling
In web applications, forms submitted via GET requests generate URIs that contain various input data. Parsing these URIs allows developers to retrieve user input easily.
Best Practices for URI Parsing
To ensure robust and secure URI parsing, developers should adhere to a number of best practices:
- Use the URL Interface: Whenever possible, utilize the built-in URL interface for easier and more reliable parsing.
- Validate Inputs: Always validate and sanitize input data coming from URIs to prevent security vulnerabilities such as injection attacks.
- Be Mindful of Edge Cases: URIs can come in many forms; always consider how to handle malformatted URIs and other edge cases.
- Consider Performance: Excessive parsing using regex can impact performance, particularly in large-scale applications. Optimize your parsing logic as needed.
Conclusion
In conclusion, mastering how to javascript parse uri is an invaluable skill for developers focused on web design and software development. Whether you're building dynamic web applications, handling external APIs, or tracking user engagement, efficient URI parsing is at the forefront. By employing the URL interface or regex, you can ensure that your applications are robust, secure, and user-friendly. Embrace these methods, and you will see your development efficiency and application performance soar.
For businesses like semalt.tools, leveraging proper URI parsing techniques directly correlates with enhanced functionality, better user experiences, and ultimately, greater success in the digital landscape. Start integrating these practices today and watch your projects thrive.